Tailoring Global Search in FilamentPHP: Role-Based Access to Notes
Learn to customize FilamentPHP's global search based on user roles and permissions, ensuring secure and role-appropriate access in your TALL Stack app.
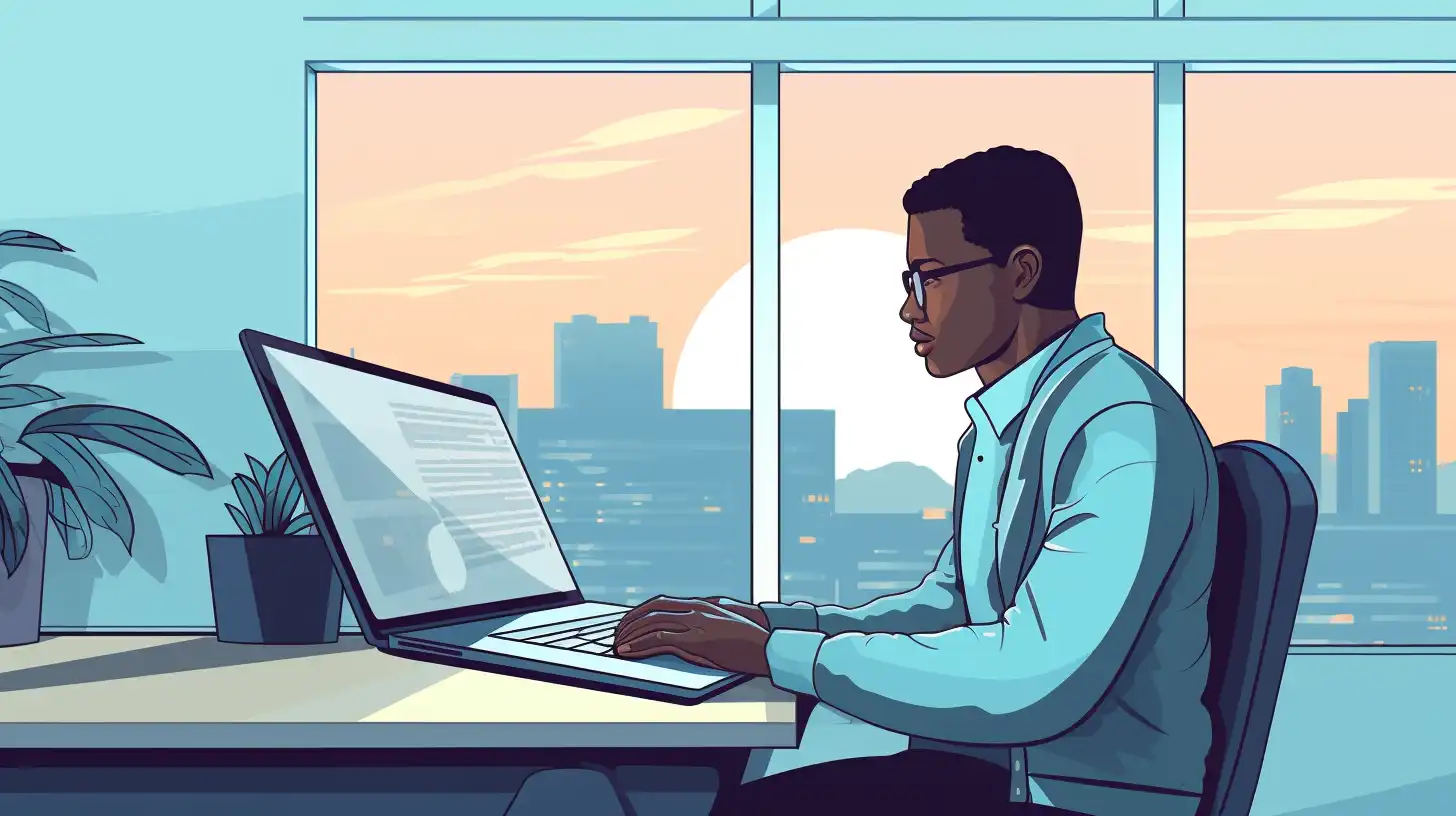
Today, I want to share a tip that I recently discovered to restrict access to a Model (Notes) via a visibility/roles relationship. I've implemented this solution in Laravel 10 using FilamentPHP overriding the FilamentPHP Global Search functionality.
Understanding the Scenario
In our application, we have two key models: Notes
and Roles
, along with a pivot table that governs access to notes based on the visibility_roles
relationship. We classify our roles into three types:
- Administrators (role_id = 1),
- Managers (role_id = 2),
- Everyone (role_id = 3).
Our goal is to ensure that only users with the appropriate role can search for specific notes. Without this restriction the application might leak sensitive information via the global search that is implemented in FilamentPHP by default.
The Magic of getGlobalSearchEloquentQuery
FilamentPHP provides a powerful method called getGlobalSearchEloquentQuery
that allows us to customize the global search query. By overriding this method, we can implement our role-based access control.
Here’s how you can do it:
public static function getGlobalSearchEloquentQuery(): Builder
{
return parent::getGlobalSearchEloquentQuery()
->whereHas('visibility_roles', function ($query) {
$query->where('role_id', RoleEnum::USER); // Filtering for notes with a role_id of 3 (Everyone)
})
->orWhere('user_id', Auth::id())
->with(['user', 'category']);
}
In this code snippet, we're modifying the global search query to include notes based on the visibility_roles
relationship. Specifically, we're filtering for notes accessible to everyone (role_id of 3) or notes that belong to the currently authenticated user.
What Does This Mean?
This implementation ensures that:
- Administrators can access notes designated for Administrators only.
- Managers can access notes meant for Administrators and Managers.
- All users can access notes designated for everyone.
Why This Matters
For newbies in Laravel 10, PHP, and FilamentPHP, understanding how to control access in your applications is crucial. By leveraging Laravel's robust authorization features and FilamentPHP’s customizable components, you can build secure, role-based applications effectively.
Conclusion
Restricting global search based on roles and permissions is a vital aspect of building secure web applications. Through this example, you've seen how seamlessly Laravel and FilamentPHP work together, harnessing the full power of the TALL stack. Keep exploring and fine-tuning your applications to make them as user-friendly and secure as possible!
Happy coding!